# Master Essential C++ Tricks to Enhance Your Programming Skills
Written on
Chapter 1: Key C++ Programming Techniques
Learn some vital C++ programming techniques that can help you write cleaner and more efficient code.
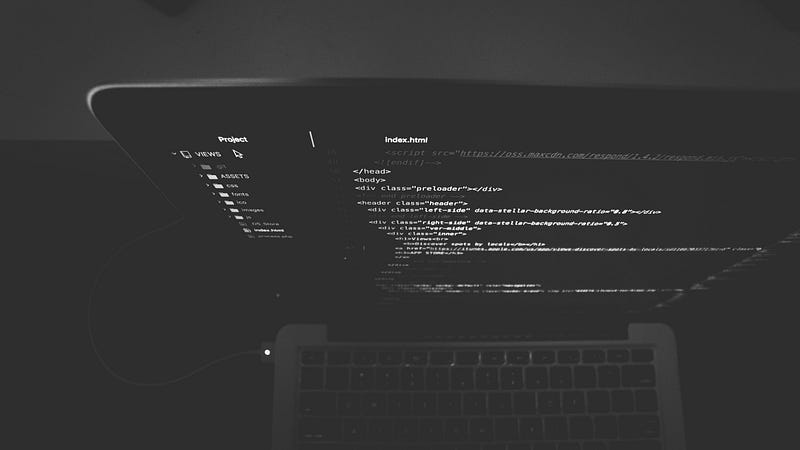
- Include All Standard Libraries at Once
Instead of including each standard library individually, you can simply use #include <bits/stdc++.h>. This is particularly handy during programming competitions where every second counts.
For instance, you can condense this:
#include <iostream>
#include <algorithm>
#include <vector>
#include <string>
#include <stack>
#include <set>
#include <queue>
#include <map>
Into this single line:
#include <bits/stdc++.h>
However, be cautious: <bits/stdc++.h> includes many headers that may not be necessary for your project, which could lead to longer compilation times. Additionally, it's not a standard header in the GNU C++ library, so you may encounter issues with non-GCC compilers, although this is rare.
- Utilize the Auto Keyword for Type Inference
Starting from C++11, the auto keyword allows you to declare a variable without explicitly defining its type, which can simplify your code, especially when dealing with iterators.
For example:
auto a = 'a';
auto t = true;
auto x = 1;
auto y = 2.0;
- Employ Range-Based For Loops
The range-based for loop, introduced in C++11, offers a more intuitive way to iterate through collections.
The syntax is as follows:
for (range_declaration : range_expression)
Here’s how to iterate through an array of integers:
int numbers[] = {1, 2, 3, 4, 5};
for (auto number : numbers) {
cout << number << endl;
}
You can also loop through the characters of a string similarly:
string name = "Charlie";
for (auto character : name) {
cout << character << endl;
}
- Simplify If…Else Statements
You can convert traditional if...else statements into concise one-liners using the ternary operator.
For example, the following code:
int age = 9;
if (age < 18) {
printf("A Child");
} else {
printf("An Adult");
}
Can be neatly rewritten as:
age < 18 ? printf("A Child") : printf("An Adult");
The general syntax for a ternary operator is:
condition ? true_expression : false_expression
While the ternary operator is common in many programming languages, be mindful of overusing it as it can affect code readability.
- Swap Variables Without an Extra Variable
You can use the XOR operator to swap two variables without needing a third variable. Here’s how:
int a = 1;
int b = 2;
a ^= b ^= a ^= b;
- Utilize the --> Operator for Loops
Although --> is not a true operator, it can function as a decrementing mechanism in a while loop. For instance, to print numbers from 9 to 1, you can write:
int x = 10;
while (x --> 0) {
printf("%d ", x);
}
This essentially operates as while ((x--) > 0).
- Prefer Pre-Increment Over Post-Increment
In C++, you can increment a value using either pre-increment (++i) or post-increment (i++). Pre-increment is generally more efficient because it doesn’t create a copy of the variable.
- Combine Assignment with Function Calls
In C++, you can streamline your code by combining assignment with function calls. Instead of writing:
int i;
i = 10;
printf("%d", i); // prints 10
You can condense it to:
printf("%d", i = 10); // prints 10
That's a wrap on these essential C++ programming tricks!
The first video, titled "How I Program C," provides insights into practical C programming techniques that can be beneficial for learners.
The second video, "C Language Tutorial for Beginners (With Notes)," is a great resource for those starting out with C programming, offering foundational concepts and notes.
Thanks for reading! I hope you find these tips helpful!