Mastering Key Filtering in Objects Using Lodash
Written on
Chapter 1: Introduction to Lodash Key Filtering
In certain scenarios, you may wish to extract specific properties from an object and create a new object that contains only those selected keys using Lodash. This article will explore how to achieve this by employing the pickBy method.
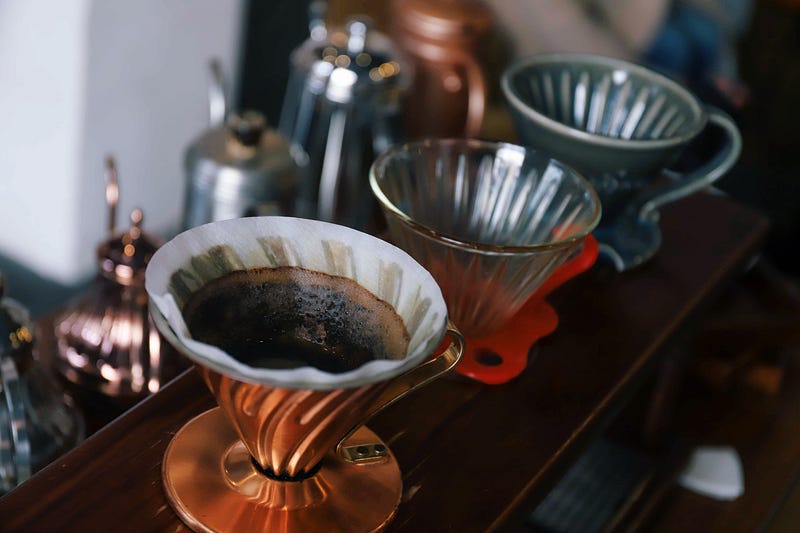
Section 1.1: Utilizing the pickBy Method
Lodash offers a handy method called pickBy, which takes an object and a callback function. This callback allows us to define the criteria for the keys we wish to retain.
For example, consider the following code:
const thing = {
"a": 123,
"b": 456,
"abc": 6789
};
const result = _.pickBy(thing, (value, key) => {
return _.startsWith(key, "a");
});
console.log(result);
In this instance, we create an object that includes only the properties from thing that begin with the letter 'a'. The first argument for pickBy is the object from which you want to extract properties, while the second argument is the callback function that specifies the conditions for the keys to be preserved.
The output will be:
{
"a": 123,
"abc": 6789
}
Section 1.2: Implementing the omitBy Method
Alternatively, you can use the omitBy method in Lodash to achieve a similar outcome. Here’s how you can do it:
const thing = {
"a": 123,
"b": 456,
"abc": 6789
};
const result = _.omitBy(thing, (value, key) => {
return !_.startsWith(key, "a");
});
console.log(result);
The parameters for omitBy are identical to those in pickBy, but the callback here defines the condition for the keys that you want to exclude from the resulting object. Consequently, the output remains unchanged from the previous example.
Chapter 2: Conclusion
In summary, both the pickBy and omitBy methods in Lodash provide powerful ways to filter keys from an object, allowing you to return only the desired properties from the original object.
The first video, "JavaScript: How to Filter Keys of an Object with Lodash?" offers an in-depth explanation of the filtering process and demonstrates practical examples.
The second video, "JavaScript Nuggets - Dynamic Object Keys," discusses dynamic object keys in JavaScript and how they relate to filtering.
For more insights, check out PlainEnglish.io. Consider subscribing to our free weekly newsletter and following us on Twitter and LinkedIn. Join our community on Discord for further discussion!