Enhancing Rust Code: Formatting, Linting, and Documentation Tips
Written on
Chapter 1: Introduction to Rust Code Quality
This article serves as a brief exploration of the processes involved in formatting, linting, and documenting code in Rust.
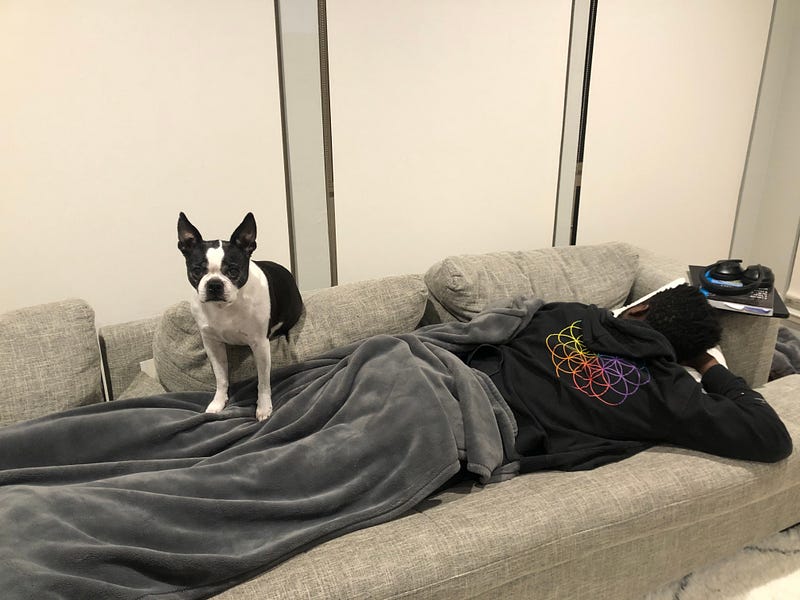
After examining some code that lacked both documentation and proper formatting or linting, I was reminded of a notable quote in the realm of software engineering: "Always code as if the person responsible for maintaining your code will be a violent psychopath who knows your address." (Attributed to John Woods)
Striving for clean and understandable code should be the top priority for every developer. When utilized effectively and consistently, tools such as formatters, linters, and documentation generators can significantly enhance code maintainability.
Following last week’s discussion about my newfound appreciation for Rust, this article will delve into the various tools available in Rust that contribute to the creation of clean code.
Section 1.1: Formatting in Rust
A formatter is designed to adjust the style of source code according to the programming language's style guidelines.
Rustfmt, distinct from the std::fmt module, is a highly configurable code formatter for Rust, controlled via a rustfmt.toml or .rustfmt.toml file.
To format your code using Rustfmt, simply execute the command cargo fmt. This command reformats the source code in your project, producing a clean output.
If you have a recent version of Rust installed, Rustfmt is likely included. If not, you can find installation instructions and more details here.
Section 1.2: Linting in Rust
Linting tools analyze code to catch potential errors, recommend best practices, and optimize existing solutions. Although the Rust compiler (cargo) is already quite stringent, Clippy, Rust’s linter, aims to highlight any suboptimal practices.
The latest version of Rust typically includes Clippy by default, but you can find installation and configuration guidance on Clippy’s GitHub page.
Consider the following Rust code, which is executable but contains some inefficiencies.
Running Clippy on this code with the command cargo clippy will produce warnings:
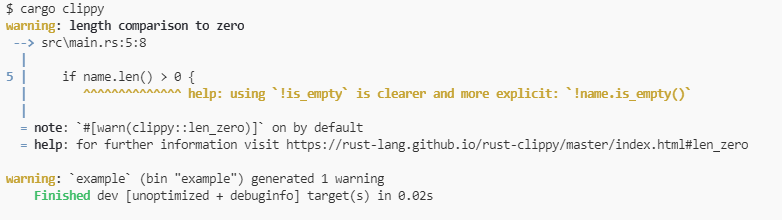
As demonstrated, Clippy effectively identifies suboptimal practices within the code and suggests better alternatives. This feature of Rust is invaluable for helping developers pinpoint potential issues and improve their coding practices.
In this video, LazyVim: Linting and Formatting, we explore how to effectively utilize linting and formatting tools in Rust.
Section 1.3: Documentation in Rust
Documentation plays a crucial role in explaining a project’s features, guiding users on how to interact with them, and easing the onboarding process.
Rustdoc is the standard tool for generating documentation in Rust projects. You can create and view documentation using the command: cargo doc --open.
But how does Rustdoc determine which parts of the source code to include? Document comments provide the answer. To create a document comment, simply begin a line with three slashes (///). This comment will only apply if it precedes an item; otherwise, you can use inner documentation comments starting with //! for parent items.
Here’s an example of Rust code featuring document comments along with the documentation generated by Rustdoc.
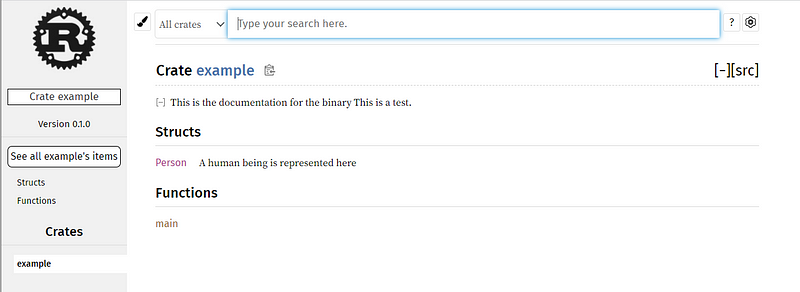
The documentation produced by rustdoc can also showcase code examples, which can be tested during your project’s testing phase (a topic I plan to cover in a future article).
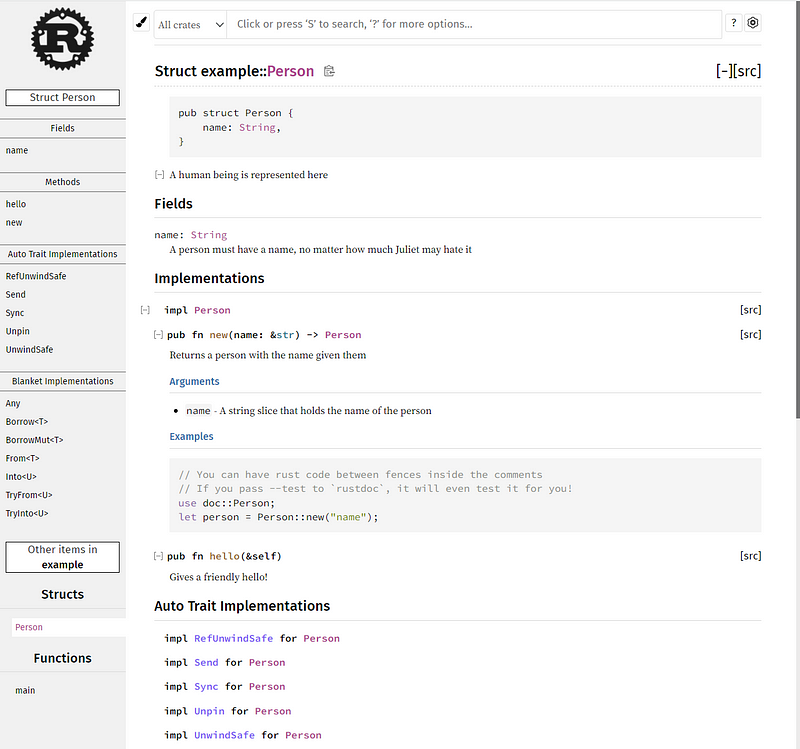
Conclusion
As we have seen, Rust provides an array of tools that can aid in producing optimal and clean code. Moreover, you can streamline your workflow by employing these tools within a Git pre-commit hook, ensuring that all code submitted for review meets minimum quality standards.
Next week, I plan to shift gears and discuss ageism in the tech industry. I have many insights on this topic, especially after sharing a relevant tweet a few weeks ago, and with my birthday approaching this week.
Wishing you all a fantastic week ahead! Your comments, applause, and follows are greatly appreciated!
Additional Resources
- Git pre-commit hook: more details here.
- Rust documentation: further information available here.
In this tutorial, Linting Rust Code With Clippy CLI Rules, we learn how to effectively use Clippy for linting Rust code, enhancing our coding practices.