Unlocking Efficient Email Management in Django with Django-Pony-Express
Written on
Chapter 1: Introduction to Django-Pony-Express
Django-Pony-Express is a groundbreaking solution for managing emails within Django applications. This innovative package adopts a class-based methodology similar to Django’s class-based views, enhancing the way emails are created, sent, and tested.
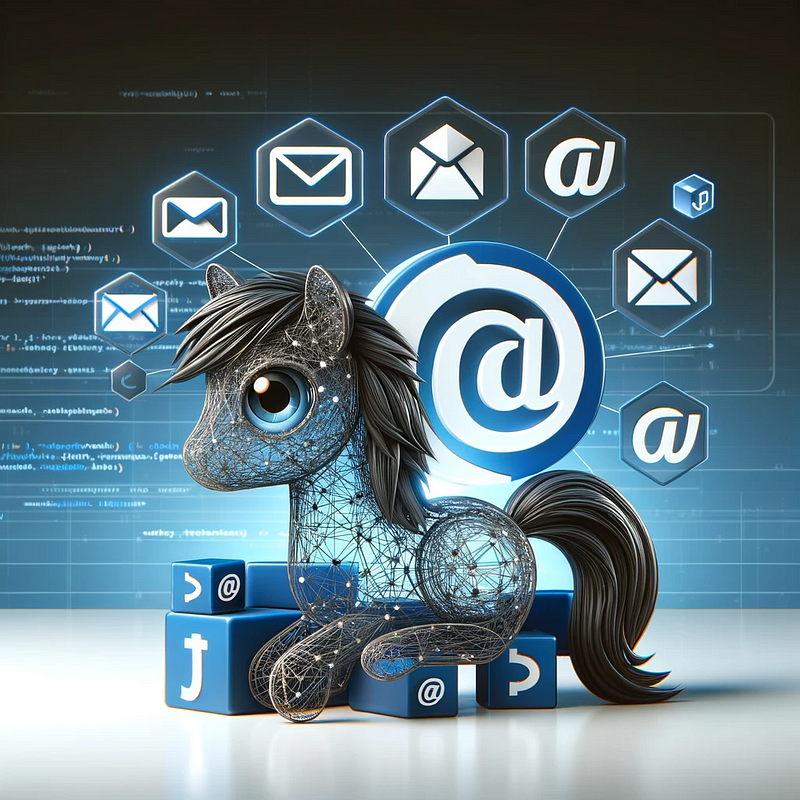
By employing this structured approach, Django-Pony-Express streamlines email handling, making it more efficient and adhering to the DRY (Don’t Repeat Yourself) principle. This enhancement not only simplifies the process but also aligns with Django’s overarching philosophy of facilitating web development.
What is Django-Pony-Express?
Django-Pony-Express is a cutting-edge email management package designed for the Django framework, enabling developers to utilize a class-based system for handling emails. This innovation aims to improve the efficiency, organization, and maintainability of email processes within applications.
Key Features
- Class-Based Design: The package utilizes a class-oriented structure for email handling, moving away from the traditional function-based methods. This allows developers to apply object-oriented programming (OOP) principles, resulting in cleaner and more reusable code.
- Adherence to DRY Principle: One of the standout benefits of Django-Pony-Express is its commitment to reducing repetitive coding, particularly when setting up email configurations and templates for various formats.
- Streamlined Email Management: By encapsulating email functionalities within classes, this package simplifies the management of all email-related tasks, from creation to customization and dispatch.
- Robust Testing Framework: Django-Pony-Express provides an extensive testing suite tailored for email functionalities, making it easier for developers to write and run unit tests, ensuring reliability and performance.
- Increased Productivity: The combination of a class-based structure and a thorough testing framework significantly minimizes the time and effort needed for email management in Django projects.
Practical Applications
Django-Pony-Express is especially beneficial in scenarios where email communication plays a vital role, such as sending transactional notifications or marketing communications, providing a systematic way to manage these tasks within the Django environment.
Installation Instructions
To install Django-Pony-Express, simply execute the following command in your terminal:
pip install django-pony-express
Next, integrate it into your Django project by adding it to the INSTALLED_APPS section of your settings file. Open your settings.py and include 'django_pony_express' in the list like this:
INSTALLED_APPS = [
# ... other installed apps ...
'django_pony_express',
]
Once these steps are completed, Django-Pony-Express will be fully operational within your project, ready for you to leverage its class-based email services.
Chapter 2: Creating and Sending Emails
Django-Pony-Express facilitates the creation of emails through a class-based structure, making the process more efficient and organized.
Here’s a straightforward example of how to create a single email class:
from django_pony_express.services.base import BaseEmailService
class MyFancyClassBasedMail(BaseEmailService):
"""
Send an email to my admins to inform them about something important.
"""
subject = _('Heads up, admins!')
template_name = 'email/my_fancy_class_based_email.html'
def get_context_data(self):
data = super().get_context_data()
data['my_variable'] = ...
return data
# Usage
from django.conf import settings
email_service = MyFancyClassBasedMail(settings.MY_ADMIN_EMAIL_ADDRESS)
email_service.process()
This example illustrates how the class-based structure simplifies the setup and dispatch of emails.
The video titled "Automating Confirmation Emails | Real Django Website | Episode #2" dives deeper into effectively automating email processes using Django-Pony-Express.
Creating Multiple Emails Efficiently
For scenarios requiring numerous similar emails, Django-Pony-Express offers a factory feature that streamlines the creation process.
class MyFancyMail(BaseEmailService):
subject = _('I am a great email!')
template_name = 'email/my_fancy_email.html'
class MyFancyMailFactory(BaseEmailServiceFactory):
service_class = MyFancyMail
def __init__(self, action_id: int, recipient_email_list: list = None):
super().__init__(recipient_email_list)
self.action = Action.objects.filter(id=action_id).first()
def get_recipient_list(self) -> list:
return self.action.fetch_recipients_for_this_action()
def get_email_from_recipient(self, recipient) -> str:
if isinstance(recipient, User):
return recipient.emailreturn recipient
def is_valid(self):
if not self.action:
raise EmailServiceConfigError('No action provided.')return super().is_valid()
def get_context_data(self):
context = super().get_context_data()
context.update({'action': self.action})
return context
This factory class adeptly manages the creation of multiple emails with tailored content for each recipient, showcasing the flexibility of the class-based approach.
Testing Email Functionality
Django-Pony-Express also provides a comprehensive framework for unit testing emails, ensuring they perform as expected across various scenarios.
To begin testing, initialize the class in your test case:
from django_pony_express.services.tests import EmailTestService
from django.test import TestCase
class MyTestClass(TestCase):
@classmethod
def setUpTestData(cls):
cls.email_test_service = EmailTestService()
This setup allows for the use of the email service across your tests, facilitating the creation of a base test class for multiple test cases.
Querying Emails
The test service can query the email outbox for specific criteria, providing a user-friendly experience akin to Django's design.
# Retrieve emails with a specific subject
my_email = self.email_test_service.filter(subject='Nigerian prince')
# Use regular expressions for filtering
my_email = self.email_test_service.filter(subject=re.compile('Ni(.*) prince'))
# Filter by recipient
my_email = self.email_test_service.filter(to='[email protected]')
Assertion Helpers
Django-Pony-Express includes various assertion helpers that streamline email testing, allowing you to verify the presence or absence of specific content in the email body.
# Assert the presence of a specific string
self.email_test_service.filter(to='[email protected]')[0].assert_body_contains('inheritance', msg='Missing words!')
# Assert the absence of a string
self.email_test_service.filter(to='[email protected]')[0].assert_body_contains_not('scam')
These helpers enhance the testing process by enabling detailed checks on email content and properties.
Conclusion
Django-Pony-Express is a game-changer for email management in Django applications, introducing a class-based framework that aligns with Django’s principles of efficient coding. This approach simplifies email handling, making it more organized, reusable, and scalable. Its commitment to the DRY principle minimizes redundancy and streamlines email setup, particularly valuable for larger projects.
Additionally, the robust testing suite ensures the reliability of email functionalities, which is essential for maintaining high communication standards. By boosting developer productivity and allowing a focus on creative aspects, Django-Pony-Express is an indispensable tool for modern Django projects, optimizing workflows and enhancing email service quality.
Check out other articles that might interest you:
- Build a Reactive ToDo application in Django with Django-Unicorn
- How to quickly expose webhooks in Django
- Mastering Object-Level Permissions in Django with Django-Rules
If you want to stay updated when I post a new article, you can sign up for my free newsletter!